56
Fetching Yelp API via Netlify Function with React.js [pt. 7]
Previous part covering steps 21-23 is right here.
In this part I will:
- fix three functionality issues (browser reload, new search, incomplete search)
-
style fetched data with
grid
,flex
andposition
properties, - deploy app to Netlify
Now, when data is securely fetched from API and correctly displayed in the browser, it is time to get rid of a few tiny functionality flaws. The following three bother me the most:
π‘ USE CASE:
I've had entered and submitted some search criteria, e.g.
pizza/Berlin
and I'm under URL withsearch?term=pizza&location=Berlin
query string. My browser shows me the rendered list of pizzerias in Berlin.entering
state is emptied ({food: "", town: ""}
).submitting
state contains{food: "pizza", town: "Berlin"}
.KEY STEP: I'm reloading the browser.
Render is gone (as I wanted and expected), so I can see only
Input
fields andSearch
button.submitting
state is emptied ({food: "", town: ""}
), that's correct too.
π΄ PROBLEM:
-
I am still under URL with
search?term=pizza&location=Berlin
query string. - I want to be under just homepage URL, without query string.
π’ SOLUTION:
π In step 12.4 (part 3) I used useHistory
hook and history
object to generate query string. I did it in Inputs
component. Right know, in this step, I'll use the same method in App
component to remove it:
π In step 16.1 (part 4) I created netlify.toml
configuration file with some redirects rules. Right now I need to update them with this code:
π I repeat the whole USE CASE, and after reloading the browser, query string is gone.
π‘ USE CASE:
I repeat steps 1-3 from previous use case
KEY STEP: without clicking at
homepage
link, nor without reloading the browser, I'm entering some new search criteria, e.g.vegan/Sydney
and submitting them.as a result of this new search, my
submitting
state contains{food: "vegan", town: "Sydney"}
as it shouldalso query string is correct:
search?term=vegan&location=Sydney
π΄ PROBLEM:
- browser still shows the same render with pizzerias in Berlin
π’ SOLUTION:
π First of all, I wanna check if API was even fetched:
So I repeat the whole USE CASE and after submission of vegan/Sydney
I check my DevTools:
Seems to me, that for some reason a new fetching wasn't triggered at all.
π To fix that, I need to make use of Dependency Array in my useEffect
hook. In step 7.2 (part 2) I passed submitting
state, as prop, to Fetched
component. Now it's time to use it:
Right now, every time when submitting
state will change, fetchingYelpFx
function will run, which means fetching will be triggered. I repeat the whole USE CASE again and after submission of vegan/Sydney
I check my DevTools:
Looks promising, so I check my render:
The currently rendered data matches the actual query string.
π‘ USE CASE:
- I search for
pizza
, but I do not specify any town. Or, I search forBerlin
, but I do not specify any food. Or, I do not specify anything at all, just clickSubmit
button.
π¨ /Berlin
SCENARIO:
-
submitting
state contains{food: "", town: "Berlin"}
-
query string contains
search?term=&location=Berlin
π¨ pizza/
SCENARIO:
-
submitting
state contains{food: "pizza", town: ""}
-
query string contains
search?term=pizza&location=
π¨ /
SCENARIO:
-
submitting
state contains{food: "", town: ""}
-
query string contains
search?term=&location=
π΄ PROBLEM:
π₯ /Berlin
SCENARIO is not big deal really, I just get useless data in my render: landmarks, buildings, parks and museums mixed up with food stands, restaurants and cafeterias. Total mess.
π₯ pizza/
and /
SCENARIOS are bigger problem which must be solved: no render whatsoever, and as if that was not enough, error in browser console:
π’ SOLUTION:
π I want to define more precisely a condition when Fetched
component will be rendered:
π I need to specify under what circumstances a query string will be generated:
π Finally, I must define when exactly a fetching can be triggered:
Right now, /Berlin
, pizza/
and /
SCENARIOS will not happen.
Right now I'm rendering data in the same order as I'm fetching it: image_url
goes first, name
goes below, rating
and review_count
go next, display_address
and each title
on the end. It looks like this:

I would like to make adjustments to my code and achieve something like this:
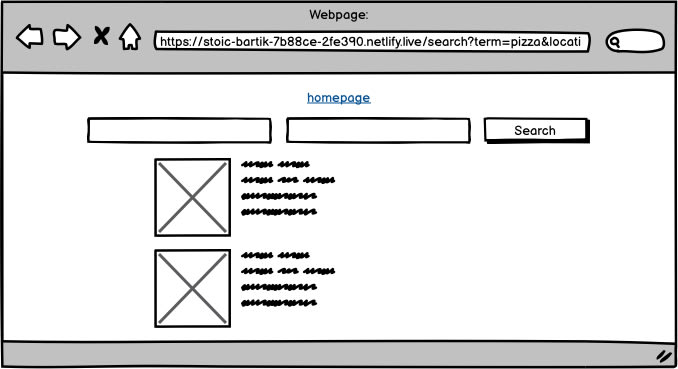
So I will:
-
add a
className
property todiv
tag containing all fetched data, -
wrap all
p
tags with text data in new, commondiv
tag withclassName
property assigned to it:
I run netlify dev --live
command, enter some search criteria, e.g. pizza/Berlin
and submit them.
With this style, for each fetched data set, I render image on the left side, and text data on the right.
Right now I'm rendering each title
below the previous one, like this:

I would like to render all of them in one line, like this:

So I will:
-
add a
className
property top
tag containing a single fetchedtitle
, -
wrap
categories
constant in newdiv
tag withclassName
property assigned to it:
With newly reorganized HTML code I can style it. For display property I will use flex value. Additionally I'll adjust some fonts size.
I run netlify dev --live
command, enter some search criteria, e.g. pizza/Berlin
and submit them.
With this style, all titles
instead of to be displayed as a list, are render in one line, like a sentence.
I would like to align each title
to the bottom of its associated image
.
In order to achieve it, I will alter default value of position property, both in div className="all-categories"
tag and his parent div className="one-single-record"
tag.
I run netlify dev --live
command, enter some search criteria, e.g. pizza/Berlin
and submit them.
titles
are aligned, seems to me style is completed.
In step 19.1 (part 5) I initialized my stoic-bartik-7b88ce
app on app.netlify.com. Since then, this app has not yet been deployed
status and to make it accessible via http
protocol, I need to run public live session with netlify dev --live
command. In the long run it's inconvenient, because each session generates random URL. I need to deploy my app to the Netlify server and make it available online under fixed URL

First action I must take to prepare my app for deployment is to use yarn build
command which generates minified production build. It works like this:
- After
yarn build
command is used, a newbuild
directory is created in my repo:
- To check what it contains, I can use this chain of three commands:
So I have one CSS file, three JS files and all of them are linked to HTML file. Entire production build is well described in CRA documentation.
After netlify deploy --prod
command is used, app is fully deployed to app.netlify.com and is available online under fixed URL.

So I go to fixed https://stoic-bartik-7b88ce.netlify.app/ URL, enter some search criteria, e.g. pizza/Berlin
and submit them.
So, my DEMO is completed, available online. I didn't implement a lot of things, such as
- data validation,
- UTF-8 encoding,
- notifications...
...because my main goals were:
- resolving CORS error,
- fetching API from Serverless Function,
- hiding API Key.
The rest of things (query string, styling) were secondary.
Complete repo from this blog entry is uploaded on my GitHub.
Thanks for reading!
56