16
Set in JavaScript: Everything you need to know
In this blog, you will learn everything you need to know about Set in javascript.
You will learn:
Suppose you want to create a list of user ids. But the list should contain unique items. How would you solve that problem?
One thing you can do is while adding an item, you can check if that item exists already in the list or not.
That will work but is not efficient. Because you have to loop over every item to check if the item exists or not. Time complexity will be O(n). Ignore time complexity if you don't know about them. Just remember it will not be efficient.
That's why we have set. Set is a data structure that only stores unique items. You can check if an item exists in the list or not very efficiently. If you are using javascript then you will not have to implement Set from scratch. Let's see how we can use Set.
We need to use the Set constructor.
const users = new Set() // Set(0) {}
const users2 = new Set([10, 12, 14]) // Set(3) { 10, 12, 14 }
You don't have to pass any arguments to the constructor. But if you pass an argument, then you have to pass iterable data. An iterable data is simply a data type that we can loop over like array and string. If you pass an array then it will remove all duplicate items. We can use this feature to remove all duplicates from an array.
const users = ['Jack', 'Mike', 'Jack', 'Roy']
// remove all duplicates
const usersSet = new Set(users) // Set(3) { 'Jack', 'Mike', 'Roy' }
// convert set to an array
const uniqueUsers = [...usersSet] // [ 'Jack', 'Mike', 'Roy' ]
That was very easy right. But what about string?
If you pass a string then the string will be split into individual characters and duplicate characters will be removed.
const name = 'Anjan'
const nameSet = new Set(name) // Set(4) { 'A', 'n', 'j', 'a' }
Simply it checks the references. The set does not contain any values. It only contains the references of the values. If the reference refers to a primitive value(number, string) then it checks with the value. Because primitives are passed by value. But if the reference is a non-primitive type then the set will compare the two references. If both reference matches, then both values will be considered as equals.
To check if an item exists in the set, we need to use the
has
method.Let's see some examples
const user = { name: 'Anjan' }
const set1 = new Set([1, 2, 3, user]) // Set(4) { 1, 2, 3, { name: 'Anjan' } }
set1.has(2) //true
set1.has({ name: 'Anjan' }) // false because this object refering to different data
set1.has(user) // true because both references are equal.
Let's see some operations with a set.
const mySet1 = new Set()
mySet1.add(1) // Set [ 1 ]
mySet1.add(5) // Set [ 1, 5 ]
mySet1.add(5) // Set [ 1, 5 ]
mySet1.add('some text') // Set [ 1, 5, 'some text' ]
const mySet1 = new Set([1, 2, 3, 4, 5, 6])
mySet1.delete(5)
mySet1.delete(2)
const mySet1 = new Set([1, 2, 3, 4, 5, 6])
mySet1.clear() // Set(0) {}
const mySet1 = new Set([1, 2, 3, 4, 5, 6]) // 6
Let's see how we can iterate over the set.
const mySet1 = new Set([1, 2, 3, 4, 5, 6])
mySet1.forEach(function (value) {
console.log(value)
})
for (let item of mySet1) console.log(item)
for (let item of mySet1.values()) console.log(item)
Note:
keys
method returns a new iterator object that yields the values for each element in the Set object in insertion order.So, that's it for today.
I have made a video about how to build a carousel postcard with React, Material-UI, and Swiper.js. If you are interested you can check the video.
You can also demo the application form here
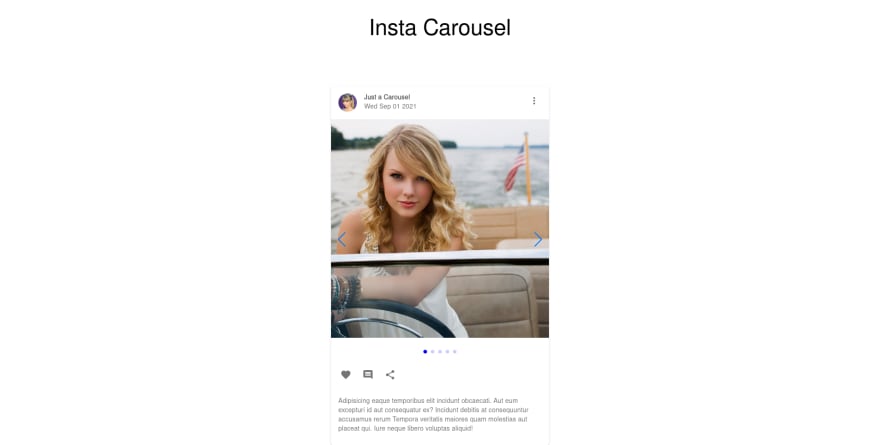
Please like and subscribe to Cules Coding. It motivates me to create more content like this.
If you have any questions, please comment down below. You can reach out to me on social media as @thatanjan. Stay safe. Goodbye.
The Internet has revolutionized our life. I want to make the internet more beautiful and useful.
I ended up being a full-stack software engineer.
I can develop complex full-stack web applications like social media applications or e-commerce sites.
I have developed a social media application called Confession. The goal of this application is to help people overcome their imposter syndrome by sharing our failure stories.
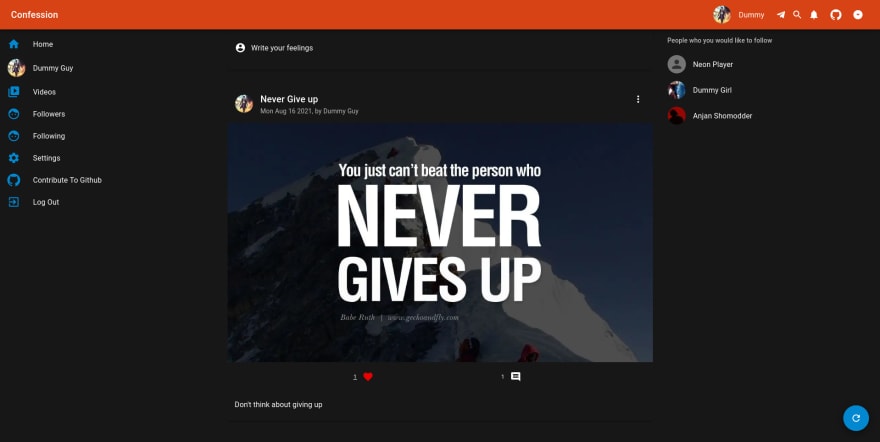
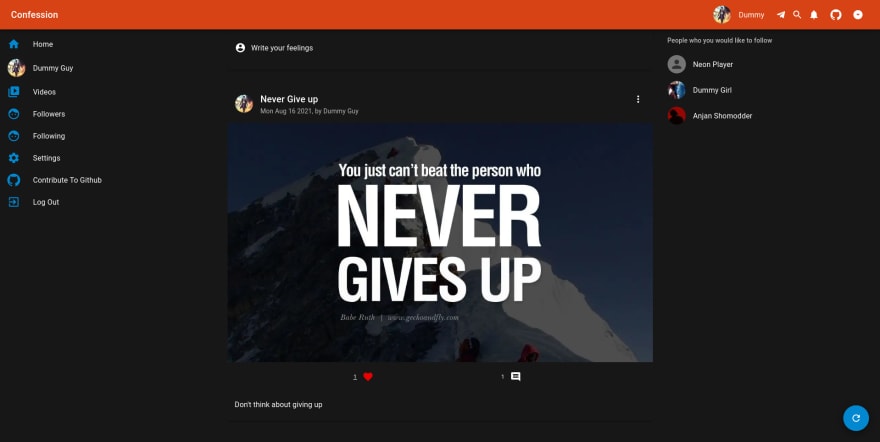
I also love to share my knowledge. So, I run a youtube channel called Cules Coding where I teach people full-stack web development, data structure algorithms, and many more. So, Subscribe to Cules Coding so that you don't miss the cool stuff.
I am looking for a team where I can show my ambition and passion and produce great value for them. Contact me through my email or any social media as @thatanjan. I would be happy to have a touch with you.
Blogs you might want to read:
Videos might you might want to watch:
16