23
How I structure my React.js projects
Structuring React applications into files and folders is an opinionated topic, because there is no right or wrong way to do it. I decided to share how I have structured my projects lately.
Warning: Highly opinionated!
Let's get started.
React projects usually start with an src/
folder and one src/App.tsx
file with an App component.

At some point, your app has more features, more lines, and you will need to make smaller standalone components:
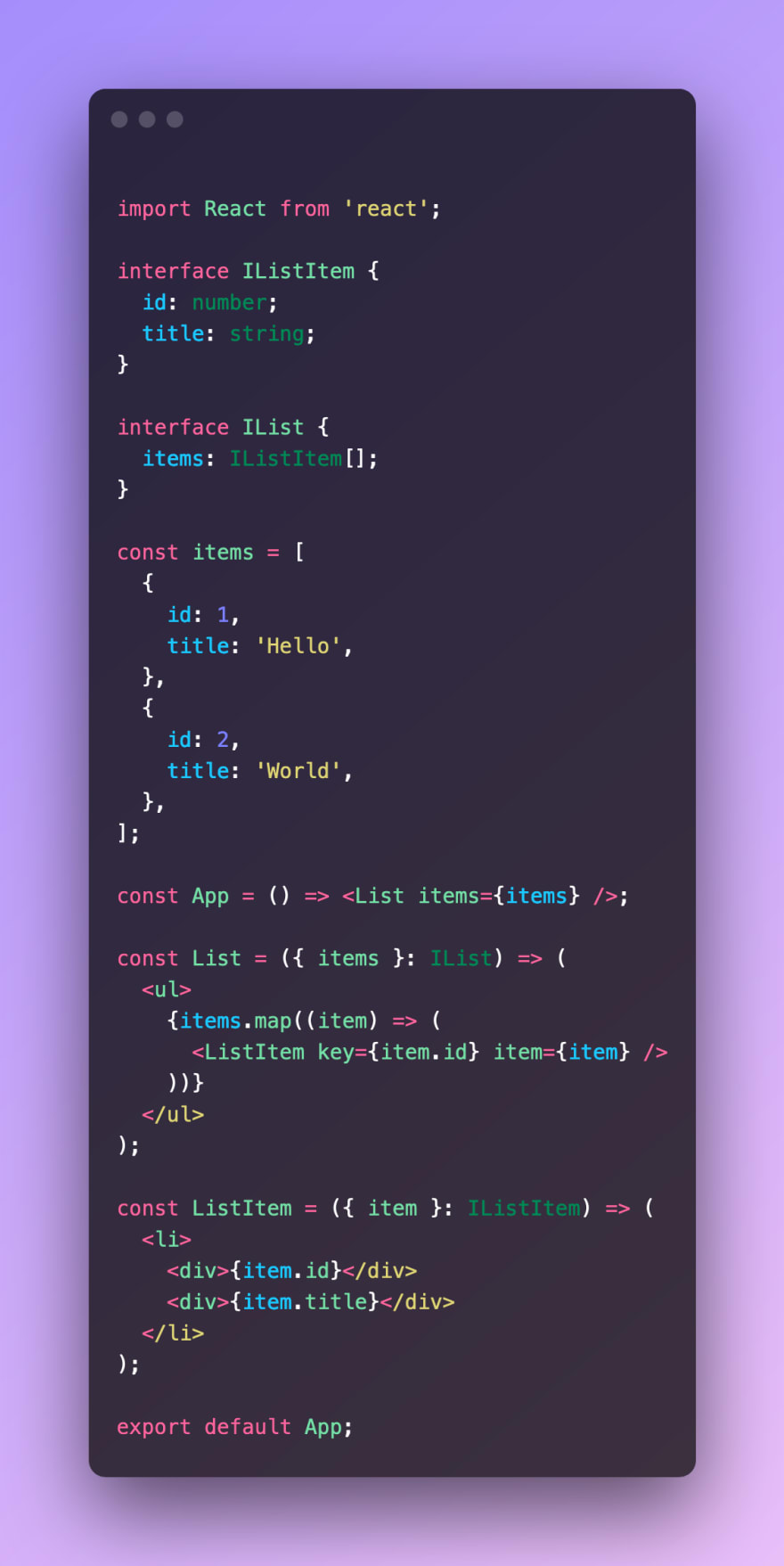
Sometimes it's OK to have multiple components in one file, especially if those components are tightly coupled to the main component. Often it's better to use multiple files because eventually, that one file is not sufficient anymore.
Instead of having all our code in one file, we can split these components into multiple files. At this point, as our project is still quite small, I would do it like this:
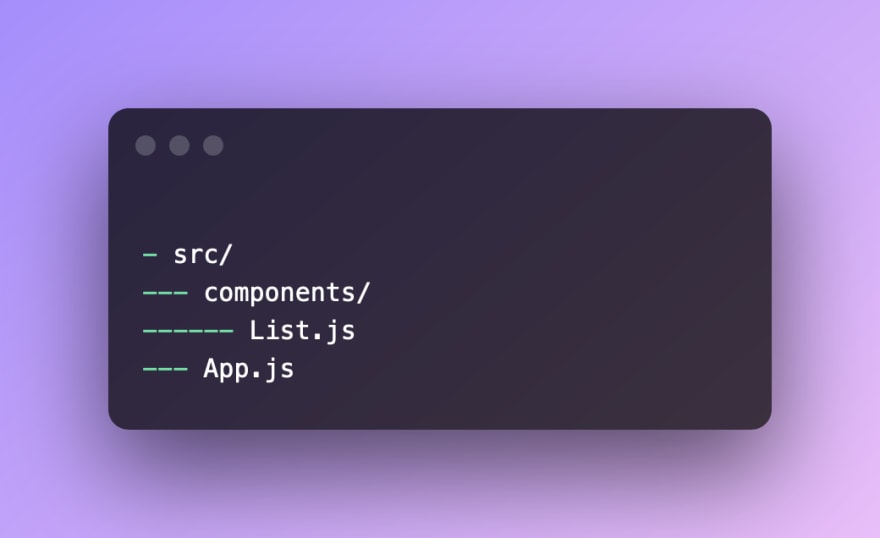
In the above example, List component exports only List
, but it also has the ListItem
component, which is not exported.
If you want to take it to the next level, you can start using directories instead of files. I would also extract the ListItem
from List
and make it its own standalone component inside src/components/List/partials
subdirectory:
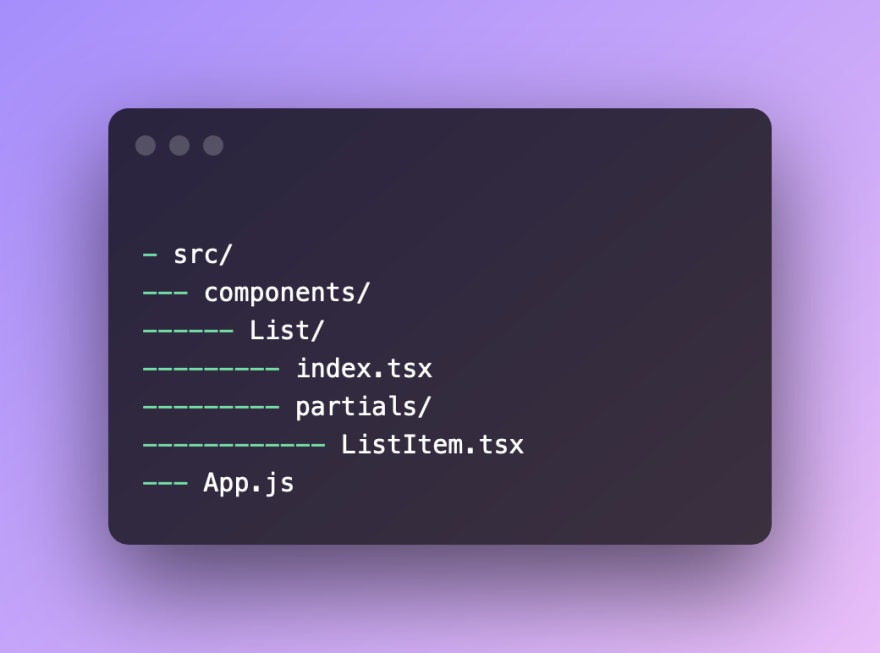
From now on, I would only use /components
directory for reusable components.
Next, we separate features from components. Let's say we need to create an useUser
hook, so we'll create a dedicated hooks
directory and put it in there:
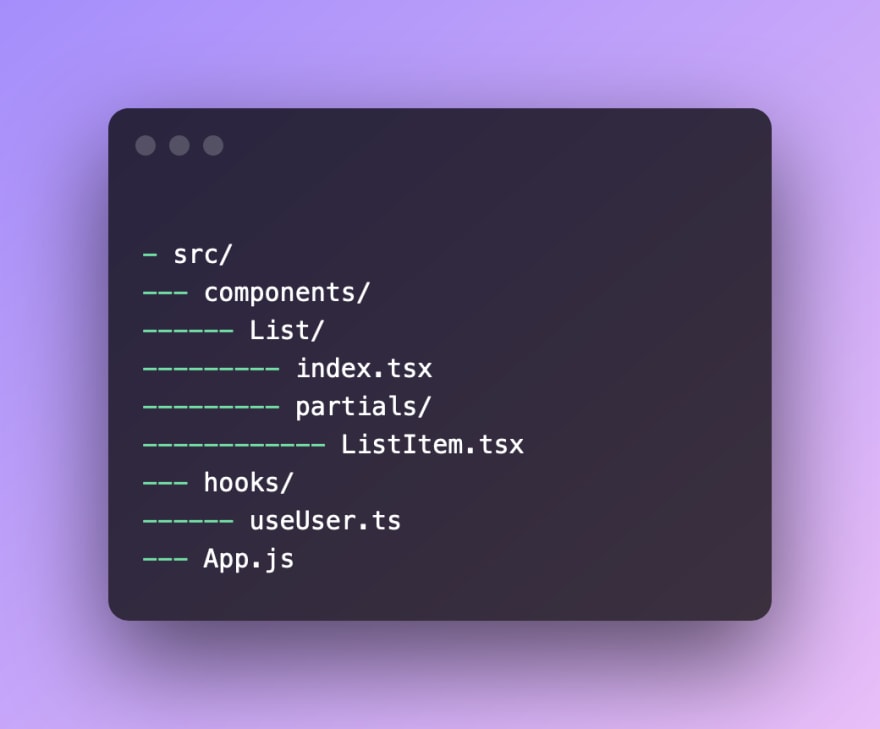
That hooks
folder is meant for reusable hooks only. If you have a hook that is used by one component only, it should remain in the component's own file (or directory).
That same principle applies to many other things as well. For example, if we need to create a reusable service - we'll create a services
directory and put our service in there:

When we create separate directories for everything that's reusable and keep the non-reusable stuff only where it is used, we've come a long way.
Lastly, I want to create a directory called views
. Those views are basically a representation of the url. For example, a directory User
could mean https://<url>/user
and so on.
These views can of course import reusable services or components, but they also have their own subdirectories for non-reusable stuff.
Here's an example, how my project structure could look like:

Follow on Twitter for more: https://twitter.com/bjakyt
23