46
Custom Cursors in React
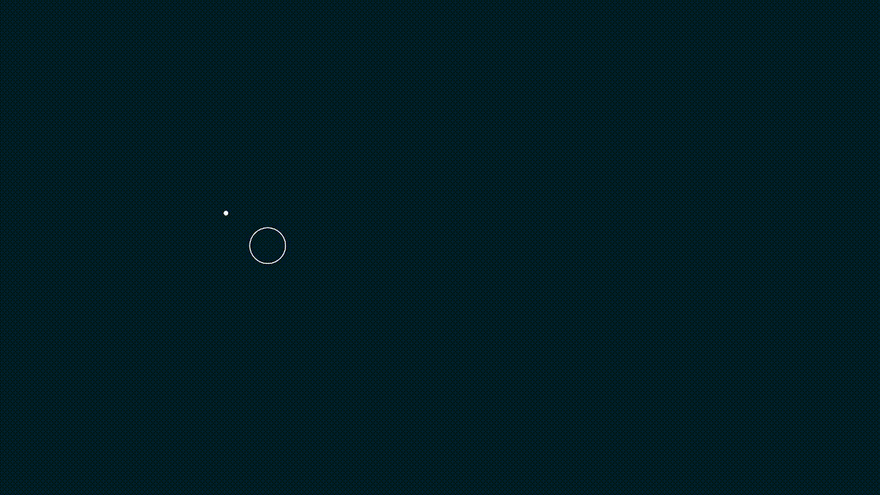
One thing I've seen across many designer/design studio websites are a cool custom cursor in place of your default computer one. Recently, I added one to my website aswell.
I had to write one from scratch (using some internet resources of course!), since all the NPM libraries I found were either outdated, or didn't support SSR.
After adding the cursor to my own website, I had an idea to create an NPM package for it for a while. This weekend, I had some freetime from school & got to work!
This library supports TypeScript, along with server-side rendering (Next.js & Remix)
Want a demo? I setup a quick demo website to help you tweak & experiment around with values!
To download the library, head over to your terminal:
yarn add custom-pointer-react
Next, import the cursor component into your file & customise it! Don't forget to wrap your app in the context afterwards.
import { Cursor } from 'custom-pointer-react'
...
const Cursor = () => {
return (
...
<Cursor {...passParametersToCustomise} />
...
)
}
...
export default App
All parameters are optional, & here's a list of the different parameters which you can customise:
Parameter | Description | Default |
---|---|---|
color | The background colour of the cursor | #000000 |
showRing | Controls whether to show the ring around the cursor | true |
ringSize | Controls the size of the ring around the cursor | 50px |
cursorSize | Controls the size of the cursor | 10px |
ringBorder | Controls the width of the ring's border | 2px |
Need help finding the right parameters? Check out the demo website!
Now, go into your app & import the MouseContext provider.
import { MouseContextProvider } from 'custom-pointer-react'
...
ReactDOM.render(
<MouseContextProvider>
<App />
</MouseContextProvider>,
document.getElementById('root')
)
...
Your custom cursor should be good to go!
46