55
Adding a REST API to your Vite Server in 5 Seconds
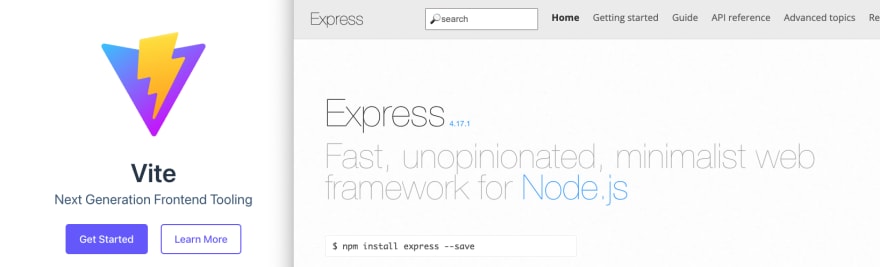
Like many others I've been blown way by the incredible performance and capabilities of vite as a tool for rapidly building out React apps. In my role as an egghead educator and a Developer Advocate at PayPal I'm looking for tools that install quickly, have minimal setup costs and let me start building immediately. vite does all that and more, but in the past I often run into one limitation: I need some kind of API to complement my apps.

I've been using express to build out APIs pretty much since it first came out. Checkout my course on using express 5 if you want a quick tutorial. Express makes it easy to build out APIs and it's super easy to add express support to your vite app. You just need a single plugin: vite-plugin-mix.
I promised 5 seconds, so get ready to copy & paste!
npm install -D vite-plugin-mix
Then in your vite.config.js
file add this to your plugins array
mix({
handler: './api.js',
}),
And then in api.js
type:
import express from 'express';
const app = express();
app.get("/api/hello", (req, res) => {
res.json({ hello: "world" });
});
export const handler = app;
Once you do that you can startup vite
with npm run dev
and like magic you'll have the ability to reference /api/hello
on your local dev server. Like everything else in vite, if you make any changes to your API they'll be available immediately without having to restart anything. Just edit the code and call the route again and you'll see the latest!
One little note: I have only used vite so far for local development and can't personally vouch for it for production apps. If you're looking for something a bit more production ready you might want to check out fastify-vite which combines another popular API server, fastify with vite for one powerful and fast full-stack development tool.
Happy hacking friends!
55