21
ECMAScript 2021 New Updates
INTRODUCTION😎
ECMAScript is a part of JavaScript language which is mostly used in web technology, building websites or web apps. ECMAScript is growing as one of the world's most widely used general-purpose programming languages. It is majorly used in embedding with web browsers and also adopted for server and embedded applications.
New updates to ECMAScript will release out this July. The new improvements are introduced to make JavaScript more powerful and also make working easy for developers. It provides new functions, simple ways to do complex works and much more.
NEW UPDATES🤩
The new JavaScript features in ECMAScript 2021 are:
1. Logical assignment operators
And & Equals (&&=)
OR & Equals (||=)
Nullish Coalescing & Equals (??=)
2. Numeric Separators
3. String replaceAll
4. Promise.any
5. Private class methods
6. Private Getters and setters
1. Logical assignment operators
Logical assignment operators introduce new operators which combine logical operators and assignment expressions.
And & Equals (&&=)
It assigns when the value is truthy.
Previous :
let x = 1;
if(x){
x = 10;
}
// Output: x = 10
New :
let x = 1;
x &&= 10
// Output: x = 10
OR & Equals (||=)
It assigns when the value is falsy.
Previous :
let x = 0;
x = x || 10;
// Output: x = 10
New :
let x = 0;
x ||= 10
// Output: x = 10
Explanation the assignment operation happens only if x is a falsy value. if x contains 1 which is a truthy value, assignment does not happen. Here x contains 0 therefore assignment happens.
Nullish Coalescing & Equals (??=)
Symbol ?? is Nullish Coalescing operator in JavaScript. It checks if a value is null or undefined.
let x;
let y = 10;
x ??= y;
Value of x is undefined, therefor the right hand side expression is evaluated and sets x to 10.
2. Numeric Separators
To improve readability and to separate groups of digits, numeric literals use underscores as separators.
// A billion dollar that I want to earn
const money = 1_000_000_000;
const money = 1_000_000_000.00;
// Also can be used for Binary, Hex, Octal bases
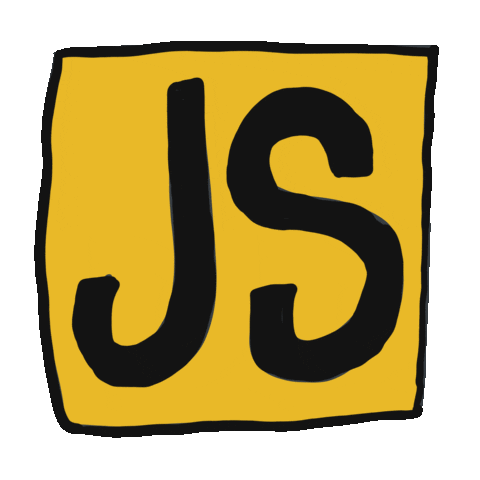
3. String replaceAll
If we want to replace all instances of a substring in string than this new method replaceAll is very useful.
const s = "You are reading JavaScript 2021 new updates.";
console.log(s.replaceAll("JavaScript", "ECMAScript"));
// output : You are reading ECMAScript 2021 new updates.
4. Promise.any
The Promise.any() method returns a promise that will resolve as soon as one of the promises is resolved. It is opposite of Promise.all() method which waits for all promises to resolve before it resolves.
Wait, what will happen when all of the promises are rejected, Yes you get that, the method will throw an AggregateError exception with the rejection reason. We have to put the code inside try-catch block.
const promiseOne = new Promise((resolve, reject) => {
setTimeout(() => reject(), 1000);
});
const promiseTwo = new Promise((resolve, reject) => {
setTimeout(() => reject(), 2000);
});
const promiseThree = new Promise((resolve, reject) => {
setTimeout(() => reject(), 3000);
});
try {
const first = await Promise.any([
promiseOne, promiseTwo, promiseThree
]);
// If any of the promises was satisfied.
} catch (error) {
console.log(error);
// AggregateError: If all promises were rejected
}
5. Private class methods
Private method have scope inside the class only so outside the class they are not accessible, see this example
Previous :
class Me{
showMe() {
console.log("I am a programmer")
}
#notShowMe() {
console.log("Hidden information")
}
}
const me = new Me()
me.showMe()
me.notShowMe()
//error
This code will throw an error that gfg.notShowMe is not a function. This is because #notShowMe() is now a private method inside the class GfG and can only be access via a public method inside the class.
New :
class Me {
showMe() {
console.log("I am a programmer");
}
#notShowMe() {
console.log("Hidden information");
}
showAll() {
this.showMe()
this.#notShowMe();
}
}
const me = new Me();
me.showAll();
//I am a programmer
//Hidden information
Now we create a new public method called showAll() inside the class Me from this public method we can access the private method #notShowMe() and since our new method is a public we get this output.
6. Private Getters and setters
Just like private methods, now we can make getters and setters as private so that they can only accessed inside class or by instance created.
class Me {
get #Name() {
return "Animesh"
}
get viewName() {
return this.#Name
}
}
let name = new Me();
console.log(name.viewName)
// Output: Animesh
Conclusion
JavaScript is one of the most popular language and these frequent updates of new features make it more awesome and development friendly. So Welcome all these new features..
Read
C++ Removed features
Updates Rocks! 😊
21