49
☢️ HTML Comment in React
If you ever started learning React and saw it's syntax JSX, you maybe thought. "This looks like HTML"
And one day you want to comment something in JSX, and your first try would be to do:
function Component() {
return (
<div>
<!-- This is my comment -->
The quick brown fox ...
</div>
)
}
And for sure your bundler started complaining, that your syntax is invalid, and then you search the internet, and realise that a HTML Comment is not valid in JSX, and you learn that you have to use a JavaScript comment.
Well in this blog post I will show you for learning purposes how to trick React to render a real HTML Comment in your browser in a few steps.
Generate a React app using Create React App
npx create-react-app my-experiment --template typescript
cd my-experiment
npm run start
Open App.tsx and add a const with a unique id as a string
const HTMLComment = 'unique-html-comment'
Declare this
HTMLComment
as a Intrinsic Element in your App.tsx. TypeScript is not required, but you have to learn something interesting 😊import { PropsWithChildren } from 'react';
declare global {
namespace JSX {
interface IntrinsicElements {
[HTMLComment]: PropsWithChildren<unknown>
}
}
}
Render this created
HTMLComment
as a JSX element in your App.tsxfunction App() {
return (
<div className="App">
<header className="App-header">
<HTMLComment>This is my comment</HTMLComment>
{/* ... */}
</header>
</div>
);
}
Let's check what was rendered in browser.

Well, that's expected, React thinks our element is a DOM element and renders it as usual. Let's move on.
createElement
function (line ~8954)} else if (typeof props.is === 'string') {
(line ~8986)
You see last
} else {
? inside that last branch a new element is created. We need to add one more if
branch to check for our HTMLComment
if (type === 'unique-html-comment') {
domElement = ownerDocument.createComment('')
}
Our final added code will look like this:
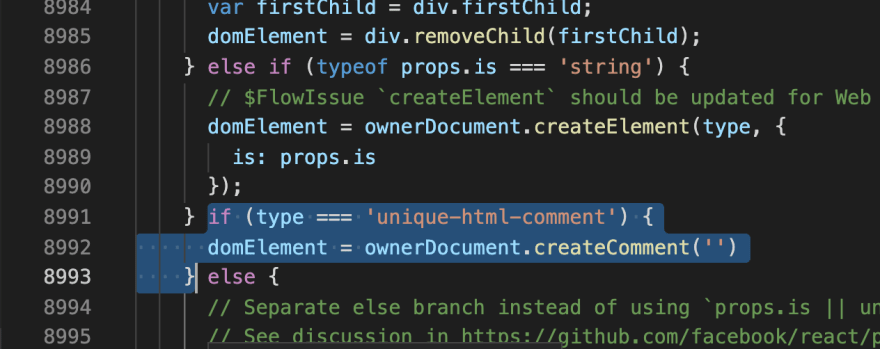
Save the file. Restart the CRA process so it can see new changes from inside
node_modules
Open the browser to see the result.

And that's how you trick React into rendering a HTML Comment!
Feeeling like a hacker now? 🤣
Feeeling like a hacker now? 🤣

Cover Photo by Florian Olivo on Unsplash
49