51
Using React useState with an object
Written by Ochuko Onojakpor ✏️
React was created to help developers easily and efficiently perform Document Object Model (DOM) manipulations in their browsers than the conventional way using vanilla Javascript.
One of React’s most commonly used Hooks is useState
, which manages states in React projects as well as objects’ states. With an object, however, we can’t update it directly or the component won’t rerender.
To solve this problem, we’ll look at how to use useState
when working with objects, including the method of creating a temporary object with one property and using object destructuring to create a new object from the two existing objects.
To understand how to manage an object’s state, we must update an item’s state within the object.
In the following code sample, we’ll create a state object, shopCart,
and its setter, setShopCart
. shopCart
then carries the object’s current state while setShopCart
updates the state value of shopCart
:
const [shopCart, setShopCart] = useState({});
let updatedValue = {};
updatedValue = {"item1":"juice"};
setShopCart(shopCart => ({
...shopCart,
...updatedValue
}));
We can then create another object, updatedValue
, which carries the state value to update shopCart
.
By setting the updatedValue
object to the new {"item1":"juice"}
value, setShopCart
can update the value of the shopCart
state object to the value in updatedValue
.
To take a step forward, we can create a function to wrap the removed logic triggered by submitting a form:
import React, { useState } from 'react';
function App() {
const [shopCart, setShopCart] = useState({item1:"Juice"});
const handleChange = (e) => {
let updatedValue = {};
updatedValue = {item1:e.target.value};
setShopCart(shopCart => ({
...shopCart,
...updatedValue
}));
}
return (
<div classname="App">
<h3>useState with object in React Hooks - <a href="https://www.logrocket.com">LogRocket</a></h3>
<br/>
<label>Name:</label>
<input type="text" name="item1" defaultValue={shopCart.item1} onChange={(e) => handleChange(e)}/>
<br></br>
<label>Output:</label>
<pre>{JSON.stringify(shopCart, null, 2)}</pre>
</div>
);
}
export default App;
By wrapping the logic we covered earlier in a handleChange
function, we can handle any changes in the input field.
Within the input field, let’s set the value of the input element to the value of item1
in the shopCart
object, which allows users to see its value as they make changes to it from the input field.
Next, let’s add the onChange
event handler to each input element, ensuring the handleChange
function triggers when we make any changes in the input field. And finally, we can display the current state of the shopCart
object as we make changes to it.
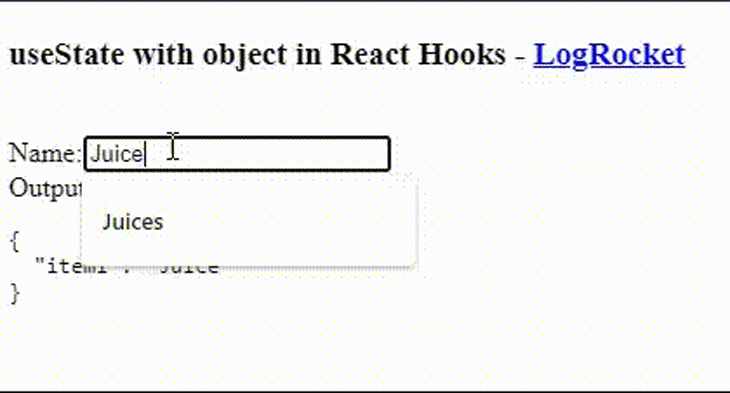
The same technique can be used to remove an item from an object:
const [shopCart, setShopCart] = useState({item1:"Juice", item2: "Icrecream"});
let copyOfObject = { ...shopCart }
delete copyOfObject['propertyToRemove']
setShopCart( shopCart => ({
...copyOfObject
}));
By creating a copy of the shopCart
state object, we can delete an item from its copy, copyOfObject
. We can then set the state of the original object, shopCart
, to the value of the copied object, copyOfObject
, using the setter object, setShopCart
, which we defined earlier.
To take a step further, we can create a function to wrap the logic, which then triggers by clicking a button:
import React, { useState } from 'react';
function App() {
const [shopCart, setShopCart] = useState({item1:"Juice", item2:"Icrecream"});
const handleClick = (item_id,e) => {
let copiedShopCart = {...shopCart};
delete copiedShopCart[item_id];
setShopCart( shopCart => ({
...copiedShopCart
}));
console.log(shopCart);
}
return (
<div classname="App">
<h3>useState with object in React Hooks - <a href="https://www.logrocket.com">LogRocket</a></h3>
<br/>
1.{shopCart.item1}
<button onClick={(e) => handleClick("item1",e)}>delete</button>
<br/>
<br/>
{shopCart.item2}
<button onClick={(e) => handleClick("item2",e)}>delete</button>
<pre>{JSON.stringify(shopCart, null, 2)}</pre>
</div>
);
}
export default App;
Again, we wrap the logic we covered earlier in the handleClick
function, which handles any click events from the buttons attached to it.
This allows us to list both items in the shopCart
object and create a button for each item.
By attaching the handleClick
function to the buttons using the onClick
event, we can pass each item’s ID in the shopCart
object to the handleClick
function to detect which item to delete when the function triggers.

This article taught you how to use useState with objects, and how to update and delete items in an object using useState
.
I recommend checking out this article to learn more about useState. If you have any questions, don’t hesitate to contact me on Twitter at @LordChuks3.
Debugging React applications can be difficult, especially when users experience issues that are hard to reproduce. If you’re interested in monitoring and tracking Redux state, automatically surfacing JavaScript errors, and tracking slow network requests and component load time, try LogRocket.
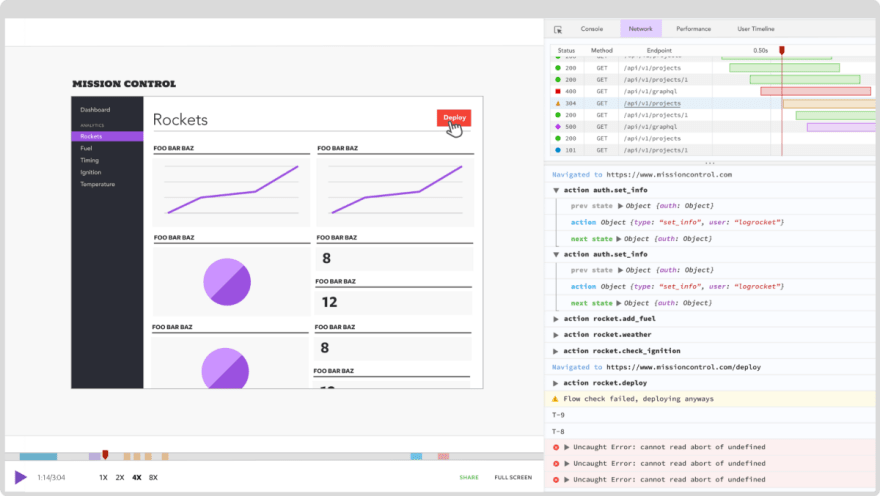
The LogRocket Redux middleware package adds an extra layer of visibility into your user sessions. LogRocket logs all actions and state from your Redux stores.
Modernize how you debug your React apps — start monitoring for free
51